C# Random与DateTime
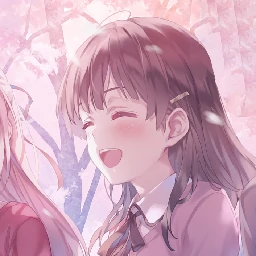
Random
表示伪随机数生成器,这是一种能够产生满足某些随机性统计要求的数字序列的算法。
创建Random实例
1 | Random random = new Random(); |
常用方法
1 | public static void Main() |
日期
DateTime
是 C# 中用于表示日期和时间的结构。它提供了丰富的方法和属性,可以用于日期和时间的计算、格式化、解析和操作。以下是对 DateTime
详细的介绍:
创建 DateTime
实例
使用构造函数
DateTime
提供了多个构造函数,可以用不同的方式来初始化日期和时间。
1 | // 使用年、月、日初始化 |
使用静态属性
DateTime
提供了一些静态属性用于获取特定的日期和时间。
1 | DateTime now = DateTime.Now; // 获取当前的日期和时间 |
常用属性
DateTime
结构提供了许多属性,可以用于获取日期和时间的不同部分。
1 | DateTime now = DateTime.Now; |
常用方法
加减时间
可以使用 Add
方法系列来对日期和时间进行加减操作。
1 | DateTime now = DateTime.Now; |
比较日期和时间
可以使用 CompareTo
方法或比较运算符进行日期和时间的比较。
1 | DateTime date1 = new DateTime(2024, 7, 20); |
格式化日期和时间
可以使用 ToString
方法和格式字符串来格式化日期和时间。
1 | DateTime now = DateTime.Now; |
时间差
可以使用 TimeSpan
结构来表示和计算两个 DateTime
之间的时间差。
1 | DateTime start = new DateTime(2024, 7, 20, 8, 0, 0); |
日期和时间的解析
可以使用 Parse
或 TryParse
方法将字符串解析为 DateTime
。
1 | string dateString = "2024-07-20 15:30:45"; |
DateTime
的限制和注意事项
最小值和最大值:
DateTime
具有静态属性MinValue
和MaxValue
,分别表示可以表示的最小和最大日期和时间。1
2DateTime min = DateTime.MinValue; // 0001-01-01 00:00:00
DateTime max = DateTime.MaxValue; // 9999-12-31 23:59:59时区:
DateTime
不直接包含时区信息。如果需要处理时区相关的日期和时间,可以考虑使用DateTimeOffset
。
时间戳的获取
1 | // 当前 UTC 时间的 Unix 时间戳: |
参考内容
参考内容:
- 标题: C# Random与DateTime
- 作者: 日之朝矣
- 创建于 : 2024-07-30 12:53:27
- 更新于 : 2024-08-18 09:25:27
- 链接: https://blog.rzzy.fun/2024/07/30/csharp-random-and-datetime/
- 版权声明: 本文章采用 CC BY-NC-SA 4.0 进行许可。
评论